Create an accordion on AMP webpage
To create an accordion in AMP you can use the HTML component amp-accordion provided by the AMP accordion script library
<!--Load amp-accordion script in the head tag-->
<script async custom-element="amp-accordion" src="https://cdn.ampproject.org/v0/amp-accordion-0.1.js"></script>
<!--Add accrodion HTML -->
<amp-accordion class="myaccordion">
<section expanded>
<h3>Accordion Header 1</h3>
<div class="content">
You can put accordion section 1 content here.
</div>
</section>
<section>
<h3>Accordion Header 2</h3>
<div class="content">Content Panel for Section 2 of the AMP accordion</div>
</section>
<section>
<h3>Accordion Header 3</h3>
<div class="content">
<amp-img src="https://placeimg.com/640/480/nature" width="640" height="480">
<div fallback>Image could not be loaded</div>
</amp-img>
</div>
</section>
</amp-accordion>
In the code snippet, we are creating an accordion that has three sections. You need to create different sections inside the amp-accordion tag. The first item of the section will become the header of the AMP accordion and the second item will become the description part for that header. The second element will show/hide on the header click.
We are creating our AMP accordion headers using the <h3> tag and the show/hide part is created using <div> element which has a class '.content'
Styling the AMP accordion
You can customize AMP accordion elements using CSS styles and apply different CSS rules to its component. We are customizing our AMP accordion using the below CSS rules and styles.
<style amp-custom>
.myaccordion {
background: #ffffff;
margin: 30px;
}
.myaccordion section h3 {
padding: 1rem;
background: #fbfbfb;
font-size: .9rem;
}
.myaccordion section .content {
padding: 1rem;
}
</style>
If you want to apply CSS on the expanded part of the AMP accordion you can use the below rule.
amp-accordion section[expanded] h3 {
color: #FF0000;
}
amp-accordion section:not([expanded]) .content {
display: none;
}
Full code to create AMP accordion
The full code that can be used to add a simple accordion on your AMP page is as below
<!doctype html>
<html amp lang="en">
<head>
<meta charset="utf-8">
<title>AMP accordion example demo - Devsheet</title>
<link rel="canonical" href="https://your_website_domain.com/page_url/">
<meta name="viewport" content="width=device-width">
<script async src="https://cdn.ampproject.org/v0.js"></script>
<script async custom-element="amp-accordion" src="https://cdn.ampproject.org/v0/amp-accordion-0.1.js"></script>
<style amp-boilerplate>body{-webkit-animation:-amp-start 8s steps(1,end) 0s 1 normal both;-moz-animation:-amp-start 8s steps(1,end) 0s 1 normal both;-ms-animation:-amp-start 8s steps(1,end) 0s 1 normal both;animation:-amp-start 8s steps(1,end) 0s 1 normal both}@-webkit-keyframes -amp-start{from{visibility:hidden}to{visibility:visible}}@-moz-keyframes -amp-start{from{visibility:hidden}to{visibility:visible}}@-ms-keyframes -amp-start{from{visibility:hidden}to{visibility:visible}}@-o-keyframes -amp-start{from{visibility:hidden}to{visibility:visible}}@keyframes -amp-start{from{visibility:hidden}to{visibility:visible}}</style><noscript><style amp-boilerplate>body{-webkit-animation:none;-moz-animation:none;-ms-animation:none;animation:none}</style></noscript>
<style amp-custom>
body {background: #f2f2f2;font-family: Arial, Helvetica, sans-serif;}
.container {max-width: 700px; margin: 0 auto;}
.myaccordion {background: #ffffff;margin: 30px;}
.myaccordion section h3 {padding: 1rem;background: #fbfbfb;font-size: .9rem;}
.myaccordion section .content {padding: 1rem;}
</style>
</head>
<body>
<div class="container">
<amp-accordion class="myaccordion">
<section expanded>
<h3>Accordion Header 1</h3>
<div class="content">
You can put accordion section 1 content here.
</div>
</section>
<section>
<h3>Accordion Header 2</h3>
<div class="content">Content Panel for Section 2 of the AMP accordion</div>
</section>
<section>
<h3>Accordion Header 3</h3>
<div class="content">
<amp-img src="https://placeimg.com/640/480/nature" width="640" height="480">
<div fallback>Image could not be loaded</div>
</amp-img>
</div>
</section>
</amp-accordion>
</div>
</body>
</html>
The above code will generate the below output.
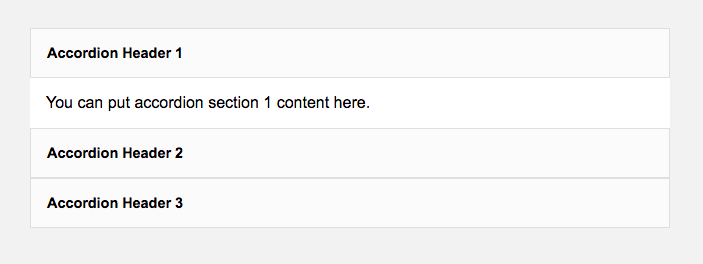