Loop through Array of Objects in PHP
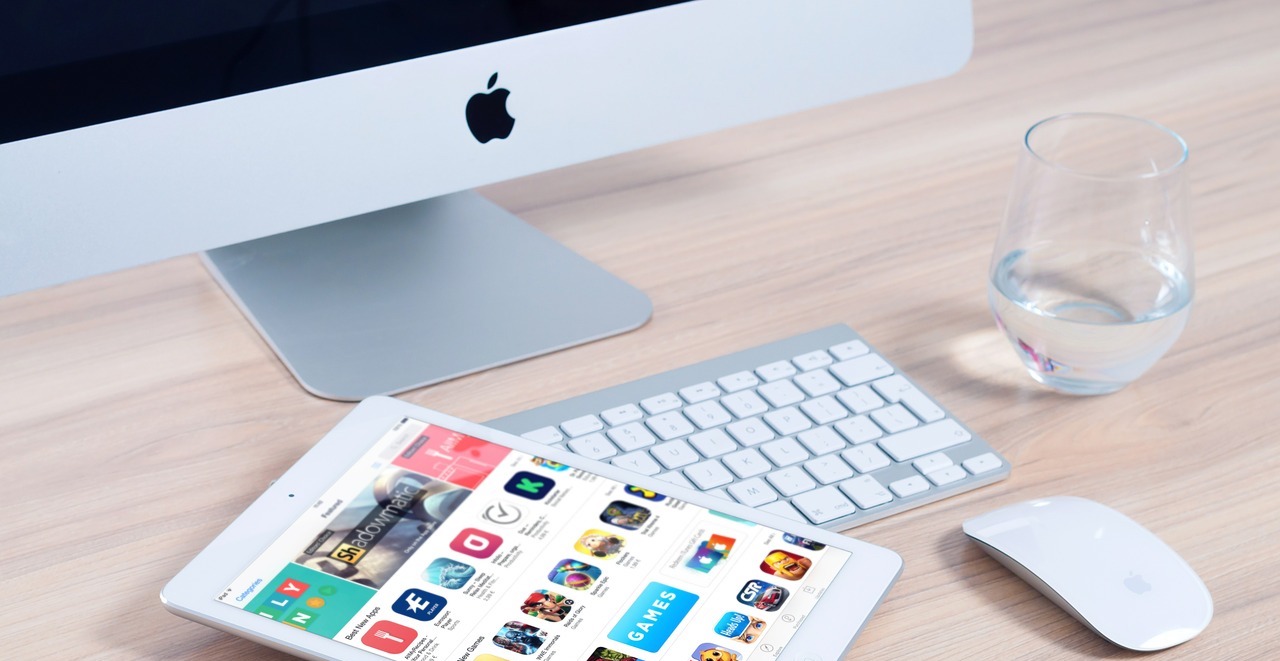
In PHP, there are a few different ways to loop through an array of objects. The most common way is to use a foreach loop. This type of loop will iterate through each object in the array and perform the specified actions.
Loop through array of objects in PHP
In order to loop through an array of objects in PHP:
- Create an array of objects. eg: $fruits = [ ["name" => "Apple"], ["name" => "Orange"] ].
- Use foreach loop of PHP to iterate over the array. eg: foreach($fruits as $key=>$value) { echo $value['name']; }.
Use PHP foreach loop to loop through Array of Objects
The PHP foreach loop is a way to iterate through an array of objects. This loop will take each object in the array and perform the specified operation on it.
The below PHP code example shows how to loop through an array of objects using the foreach loop. This is a common operation in PHP when working with arrays of data.
Code example
<?php
$students = [
["id" => 1, "name" => "John", "department" => "Science" ],
["id" => 2, "name" => "Sonia", "department" => "Arts" ],
["id" => 3, "name" => "Tony", "department" => "Science" ]
];
foreach($students as $key=>$value) {
echo "Id: {$value['id']}, Name: {$value['name']}";
echo $value['department'];
}
?>
Output
Id: 1, Name: John
Science
Id: 2, Name: Sonia
Arts
Id: 3, Name: Tony
Science
The code above is an example of a foreach loop. This loop will iterate through each element in the $students array and print out the id, name, and department for each student.
Loop through Array of Objects using For loop
Here we will describe how to use a For loop to iterate through an array of objects. This can be useful when you need to perform an action on each object in the Array, such as displaying data from the object or calling a function that uses data from the object.
<?php
$students = [
["id" => 1, "name" => "John", "department" => "Science" ],
["id" => 2, "name" => "Sonia", "department" => "Arts" ],
["id" => 3, "name" => "Tony", "department" => "Science" ]
];
for($i = 0; $i < count($students); $i++) {
echo "Name: {$students[$i]['name']}";
}
?>
Output
Name: John
Name: Sonia
Name: Tony
The code above creates an array of student information, then uses a for loop to iterate through the array and print out each student's name.
Use While loop to loop through an array of object
While loop can be used to loop through an array of objects.
While loop will iterate through each element of the array.
<?php
$students = [
["id" => 1, "name" => "John", "department" => "Science" ],
["id" => 2, "name" => "Sonia", "department" => "Arts" ],
["id" => 3, "name" => "Tony", "department" => "Science" ]
];
$i = 0;
while($i < count($students)) {
print($students[$i]['name']);
$i++;
}
?>
Output
John
Sonia
Tony
The code above creates an array of student information, then uses a while loop to print each student's name.
The while loop will continue until the variable $i is no longer less than the number of students in the array (count($students)).
Conclusion
These were some methods that can be used to iterate over an array of objects in PHP. We have explained most of them here but if you have more code examples and techniques in order to do that, you can also contribute here.