Check if Array includes String(Case Insensitive) Javascript
There are multiple methods that can be used to check whether a string exists inside an array as an item or not. But if you want to check the string ignoring if it is lowercase or uppercase then you can use the code examples explained in this post.
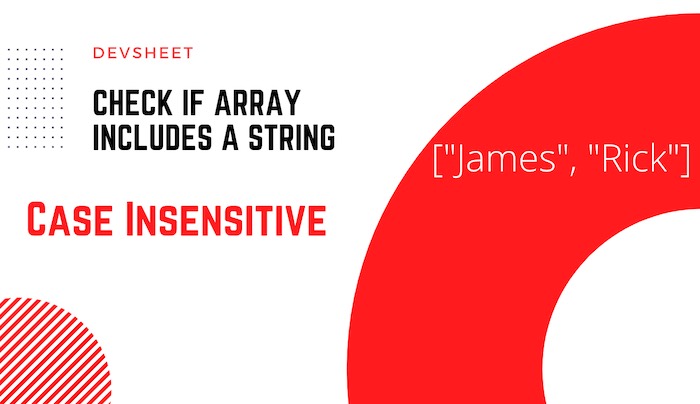
Use Array.findIndex() method to check if Array includes String(Case Insensitive)
The function will return the index of the string if the string is found in the array and -1 if not found in the array.
The simplest way to check if a string is included in an array ignoring cases is to use the Array.findIndex() function. The process of finding a string inside the array ignore case is as below.
- Create an array that contains multiple string items.
- Use Array.findIndex() function and inside this function convert the string to lowercase along with the converting array item to lowercase.
- Check the returned index.
Code example 1: Using Array.findIndex() and String.toLowerCase() functions
const shows = ["Mandalorian", "Breaking Bad", "Games of Thrones", "Sillicon Valley"];
const show = "breaking bad";
const result = shows.findIndex( item => show.toLowerCase() === item.toLowerCase());
console.log(result);
Output
1
In the above code example, we have created an array named shows that contain multiple items. Using shows.findIndex() and lowercase() function we are ignoring the case and find the string in the array and assigning the returned value to the result variable. It is returning 1 as the string found in the array.
Code example 2: Using Array.findIndex() and toUpperCase() functions
const subjects = ["Math", "Physics", "Chemistry", "English", "Economics"];
const sub = "english";
const result = subjects.findIndex(item => sub.toUpperCase() === item.toUpperCase());
if (result !== -1) {
console.log("String exists in the array");
} else {
console.log(" String des not exist in the array");
}
Output
String exists in the array
Code example 3: Create a function to check for multiple strings
function string_exists(arr, val) {
const result = subjects.findIndex(item => val.toUpperCase() === item.toUpperCase());
if (result !== -1) {
return true;
}
return false;
}
const subjects = ["Math", "Physics", "Chemistry", "English", "Economics"];
console.log( string_exists(subjects, "chemistry") ); // -> true
console.log(string_exists(subjects, "hindi")); // -> false
In the above code example, we have created a function string_exists() that takes two parameters:
arr - the array in which string needs to be found.
val - The string that is searched in the array.
The function will return true if the string is included in the array and false if not.
Convert Array values to lowercase then find the String - Case insensitive
Another solution to check if a string is included in an array - case insensitive is by converting all the values to lowercase and then performing the check.
We will be using the Array.map() and toLowercase() functions to convert all the values of our array to lowercase and then using the indexOf() function we are checking if a string is included in an array ignoring case sensitivity.
// create an array
let arr = ["Tony", "James", "Rick", "Carol", "Nambiar"];
// create a temp array with lowercase values
arr_temp = arr.map(function (item) {
return item.toLowerCase();
});
// check if 'ricK' is included in array
const val1 = "ricK"
const result1 = arr_temp.indexOf(val1.toLowerCase());
console.log(result1);
// -> 2
// check if 'Steve' is included in array
const val2 = "steve";
const result2 = arr_temp.indexOf(val2.toLowerCase());
console.log(result2);
// -> -1
In the above code example
- We have created an array named arr that contains multiple strings items in it.
- Using Array.map() and String.toLowerCase() functions, we are creating a temporary array named arr_temp that will contain all the values or arr in the lowercase format of items.
- We are testing the new array arr_temp against two values - ricK and Steve.
Use Array.some() function to check if Array includes a String - ignore case
In Javascript, the Array prototype provides a lot of functions that make arrays manipulation very easy. Array.some() function is one of them that we will use to check if a value is present in an array without considering case sensitivity(Lowercase or uppercase).
const myarray = ["Tony", "James", "Rick", "Carol", "Nambiar"];
const q = "caRoL";
const result = myarray.some( item => item.toLowerCase() === q.toLowerCase() );
console.log(result);
Output
true
In the above code example, the result variable will be true as the Array.some() function is returning value true if the string is found in the array. We are comparing the values with their lowercase version here.
Using join() and indexOf() functions
We will use join(), indexOf(), and toLowerCase() functions here to check if an array includes a string ignoring the case sensitivity.
- Create a string from the array with comma-separated values using Array.join() function.
- Convert the above string to lowercase characters using String.toLowerCase() function.
- Check the query string after converting it to lowercase also with the string created from the array. We are using indexOf() function for that.
var fruit = "Avacado"
var fruits = ["BaNana", "GraPEs", "AvaCaDO"];
var lower_strs = fruits.join(',').toLowerCase();
console.log(lower_strs)
if (lower_strs.indexOf(fruit.toLowerCase()) > -1) {
console.log("String is found in array");
} else {
console.log("String not found in array")
}
Output
banana,grapes,avacado
String is found in array
Using Array.find() function
Array.find() function is used to find an element in an array. if the item is found it will return the item else undefined. We will use Array.find() function here and compare it with undefined to return true if the string is found and return false if not.
var fruit = "Avacado"
var fruits = ["BaNana", "GraPEs", "AvaCaDO"];
var isFound = fruits.find(item => item.toLowerCase() === fruit.toLowerCase()) !== undefined;
console.log("String Found: " + isFound);
Output
String Found: true
- Check if string includes a substring Javascript (Multiple Methods)
- How to Check If an Array Includes an Object
- Convert a string to array using Array.from() in javascript
- Check if a string is Palindrome or not using Javascript
- Check if all array items are equal javascript
- Check if given value is an array in Javascript
- Methods to check empty array using javascript