Create dummy APIs using json-server node module
The node package json-server can be used to create dummy REST APIs within no time. You can install the json-server module on your local system and use those apis for testing and development purpose.
Install json-server module
This is a node module means you can install json-server using npm. You can run the below command to install the json-server globally.
npm i -g json-server
To read more about the json-server package - click here.
Create a JSON file with some data
After installing the json-server package, you need to create a JSON file that will contain an object and the key names will be treated as APIs endpoints. For example, if you want to create an API that contains users list then you can create the JSON file on your system and add some uses data.
Example. To create a JSON file in the folder json_db that exists on Desktop, run the below commands.
cd ~/Desktop
mkdir json_db
cd json_db
touch db.json
The above commands will create a db.json file in the json_db folder. You create the JSON file anywhere you want on your system.
Now open the db.json file in an Editor e.g. VS code and add the below data to it.
db.json
{
"users": [
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
},
{
"id": 101,
"name": "Rick Grimes",
"email": "[email protected]"
}
]
}
Run json-server command to start the server
After creating the JSON file go to the folder where you have created the JSON file. If you have your JSON file exists on Desktop > json_db > db.json then you can run the below command to go to the directory.
cd ~/Desktop/json_db/
Now start the server using the below command
json-server --watch db.json --port 8000
Note that we are using port 8000 here to start the API server on localhost. You can also use other ports like 3000 or 400o whatever you like. After running the above command the server will be started and you will see output like the below in your terminal
\{^_^}/ hi!
Loading db.json
Done
Resources
http://localhost:8000/users
Home
http://localhost:8000
Type s + enter at any time to create a snapshot of the database
Watching...
It means your APIs are running on the server. You can check the APIs running on the system by hitting different URLs.
Now we have the below APIs running for users
GET /users
GET /users/100
POST /users
PUT /users/100
PATCH /users/100
DELETE /users/100
http://localhost:8000/users [Get API for Users list]
[
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
},
{
"id": 101,
"name": "Rick Grimes",
"email": "[email protected]"
}
]
http://localhost:8000/users/100 [Get APIS for particular user information using id]
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
}
Add new user - POST API
If you want to add a new user to the db.json file users object then you can use the POST API for that. The postman request example is as below to send a POST request to json-server.
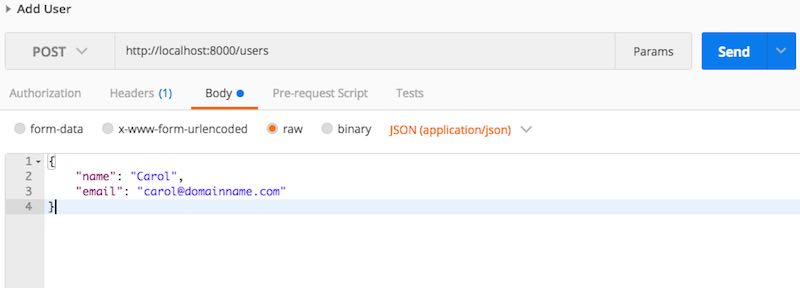
The above request will return the newly created user using http://localhost:8000/users POST API like below.
{
"name": "Carol",
"email": "[email protected]",
"id": 102
}
Now if you hit the GET API for users - you will see the below output.
GET API - http://localhost:8000/users
[
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
},
{
"id": 101,
"name": "Rick Grimes",
"email": "[email protected]"
},
{
"name": "Carol",
"email": "[email protected]",
"id": 102
}
]
Filter APIS for the 'users' endpoint
The json-server also creates filter APIs. You can use the below APIs to filter the user's data.
GET /users?name=John%20Deo
GET /users?name=John%[email protected]
GET /users?id=100&id=102
Output of http://localhost:8000/users?name=John%20Deo
[
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
}
]
Output of http://localhost:8000/users?name=John%20Deo&[email protected]
[
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
}
]
Output of http://localhost:8000/users?id=100&id=102
[
{
"id": 100,
"name": "John Deo",
"email": "[email protected]"
},
{
"name": "Carol",
"email": "[email protected]",
"id": 102
}
]