javascript
Show confirmation alert before submitting a form in Javascript
In this post, we will learn how to show a confirmation alert before submitting a form in Javascript. This is a great way to avoid accidental submissions and preserve data integrity.
document.getElementById("my_form").addEventListener("submit", function() {
if (confirm("Are you sure to submit")) {
return true;
} else {
return false;
}
});
By adding a few lines of code, you can ensure that your users confirm their submission before the form is actually submitted. In the above code example,
- We have created a form and added an Event Listener to it on the form submit.
- When the user submits the form, we are showing an alert box or confirmation box. That will have a message that you passed in the confirm() function as a parameter. Also, there will be two buttons in the alert box - cancel and ok.
- If you press the cancel button you can use return false to not to submit the form.
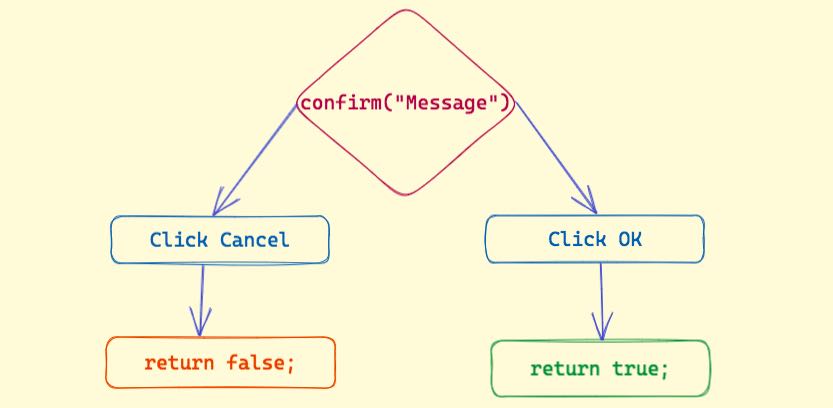
The minimal code that can be used to show the confirmation box before the form submits is as below.
if (confirm("Are you sure to submit")) {
return true;
} else {
return false;
}
If you are using jQuery, you can use the below code
$("my_form_id").submit(function() {
if (confirm("Are you sure to delete it")) {
return true;
} else {
return false;
}
});
Was this helpful?
Similar Posts
- Get text before @ in an email using Javascript
- Create a simple Login Form using Redux Form
- Call custom method on form submit redux form
- Create form data in Javascript
- xhr post request with form data javascript
- Show data values on charts created using chart.js
- Show vertical line on data point hover Chart.js