How to remove debug banner in Flutter app
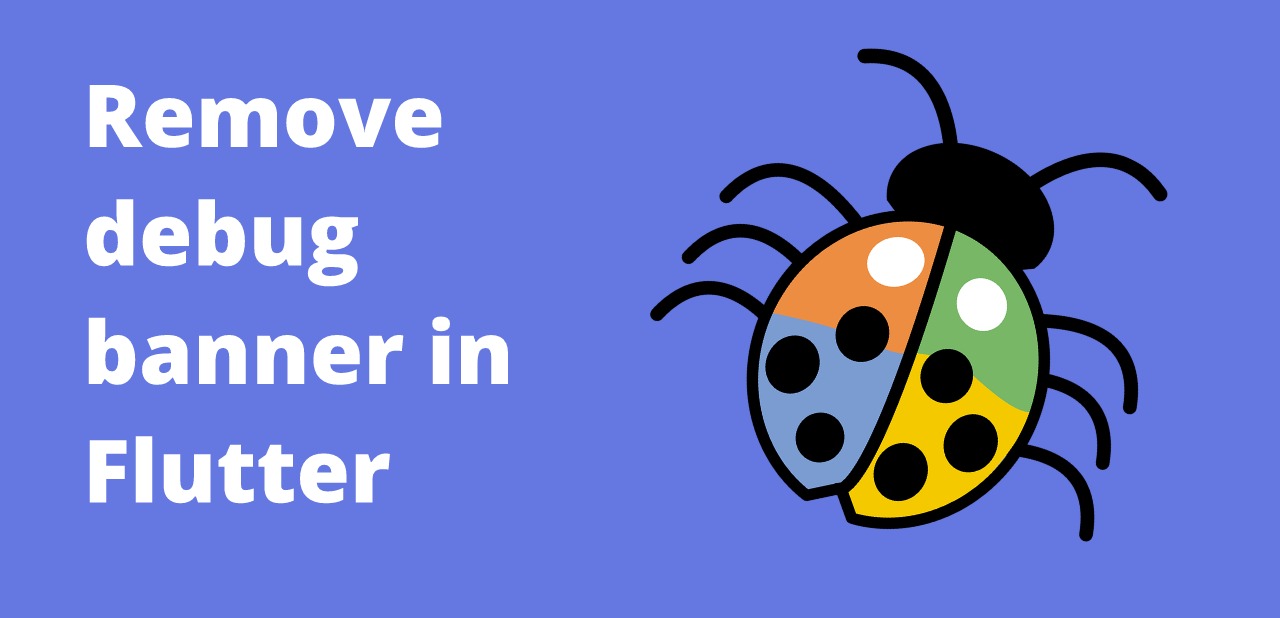
The debug banner is a visual indicator that appears in the top right corner of the screen when running a debug build of a Flutter app. While the debug banner can be helpful when developing and debugging an app, it can be annoying when running a release build of the app. This article will show you how to remove the debug banner in a Flutter app.
MaterialApp(
title: 'My App',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
How to remove debug banner in Flutter app
To remove debug banner in Flutter application you can set debugShowCheckedModeBanner: false to MaterialApp() widget property.
The code that is used to remove or hide the debug banner in the Flutter app is:
debugShowCheckedModeBanner: false,
We can use it inside the MaterialApp() widget and set debugShowCheckedModeBanner to false. Now the red color ribbon of debug text will not appear on the screen when you are debugging the Flutter app.
Full code
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
debugShowCheckedModeBanner: false,
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
If you are building your app in release mode then the debug ribbon will be removed itself as it is only present if the app is building in debugging mode.
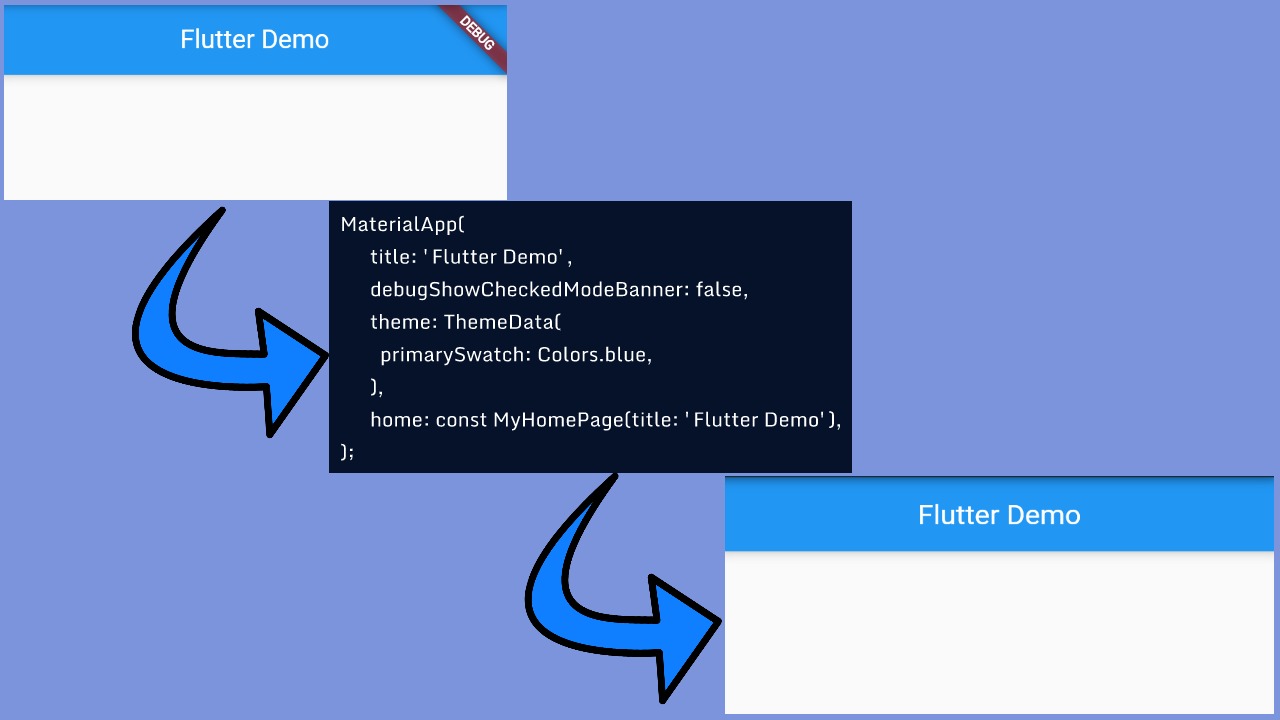
If you are using ScaffoldApp() widget then you can use the below code in your flutter app to hide the debug banner.
ScaffoldApp(
debugShowCheckedModeBanner: false,
);
# Using android studio
If you are developing the Flutter app using Android Studio IDE then you can go to:
Flutter Inspector tab > More Actions > Hide Debug Mode banner
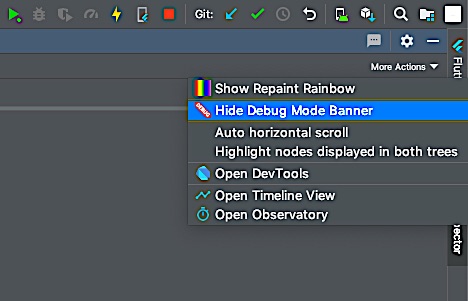