Add border to container in flutter
In order to add a border to a container in flutter, you will need to use the DecoratedBox widget. The DecoratedBox widget allows you to add a border, a background color, and a padding to a container. In order to use the DecoratedBox widget, you will need to import the material.dart package.
How to add border to container in Flutter
Adding a border to a container is a simple process:
- Create a Container Widget and add a Decoration.
- In the Decoration, specify the border using the border property.
- The border can be a solid line, a dashed line, or a dotted line.
Add a border on all sides of the Container
A border can be added to a Container in Flutter by using the decoration property. The decoration property expects a BoxDecoration, which allows you to specify the color and width of the border.
Using the below code example, we will add a border to the container widget on all sides.
Container(
width: double.infinity,
margin: const EdgeInsets.all(30.0),
padding: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
border: Border.all(
width: 2.0,
color: Colors.blue,
style: BorderStyle.solid
),
),
child: const Text('Hello world'),
),
Output
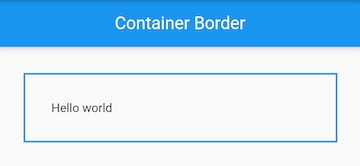
Add a border to specific sides of the Container
If you do not want to add the border on all sides of the container then you can use the below code to add the border to the Container on specific sides - left, right, top or bottom.
Container(
width: double.infinity,
margin: const EdgeInsets.all(30.0),
padding: const EdgeInsets.all(30.0),
decoration: const BoxDecoration(
border: Border(
left: BorderSide(
color: Colors.red,
style: BorderStyle.solid,
width: 2.0,
),
bottom: BorderSide(
color: Colors.black,
style: BorderStyle.solid,
width: 2.0,
),
),
),
child: const Text('Hello world'),
),
Output
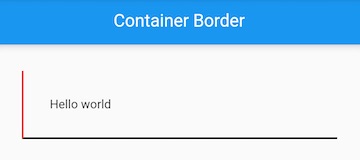
The above code will add the border to the Container on the left and bottom sides. You can also specify the top and right property to it to add the border on those sides.
We are using a solid style of the border added to the Container. We can also use dashed and dotted styles. We will read about them below.
Change the color of border of Container
We can change the color of the border by using the color property in the Border.all() or BorderSide() function. An example to apply red color to the Container border is as below:
Container(
width: double.infinity,
margin: const EdgeInsets.all(30.0),
padding: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
border: Border.all(
width: 2.0,
color: Colors.red, // change border color here
style: BorderStyle.solid,
),
),
child: const Text('Hello world'),
),
Output
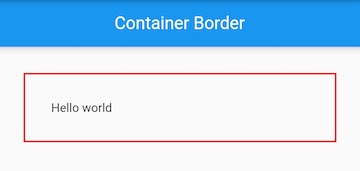
In the above code example, we have assigned a red color to our border using the color: Colors.red. You can change it according to your requirements.
Add a border with border-radius to Container
The border-radius property is used to add rounded corners to a container. We will also use a border for the container to make it more visible. An example of the same is below:
Container(
width: double.infinity,
margin: const EdgeInsets.all(30.0),
padding: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(10)),
border: Border.all(
width: 2.0,
color: Colors.grey,
style: BorderStyle.solid,
),
),
child: const Text('Hello world'),
),
Output
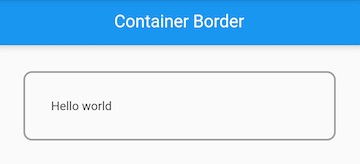
In the above code example, we have created a Container widget in Flutter. We have assigned a BoxDecoration property to it and in this property, we have added a border radius and border to the Container.
The color of the border is grey along with a border-radius 10. We have assigned width 2.0 to the border.
Conclusion
In this article, we have learned to add a border to a Container using BoxDecoration. We have also customized the border style like color, width, etc. We have explained multiple code examples that can be used to add a border to Container. If you know more about the same, You can also contribute here.