flutter
Add border radius to container in flutter
September 15, 2022
In this post, I am going to describe how to add border radius to container in flutter. This is the simplest way to add border radius to container in flutter.
Container(
margin: EdgeInsets.all(30.0),
padding: EdgeInsets.all(20.0),
decoration: BoxDecoration(
borderRadius: BorderRadius.all(
Radius.circular(10),
),
color: Colors.red,
),
child: Text(
'Hello world'
),
),
Here we are going to show you how to make rounded corners of a container or add border-radius to a container in Flutter. You can use decoration property for that and pass BoxDecoration with borderRadius property in it. We have assigned a '10.0' scale radius to the container by using 'BorderRadius.circular(10.0)'.
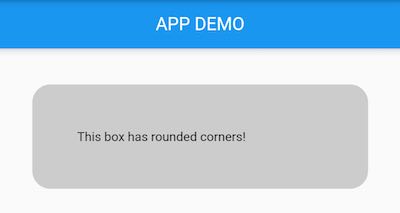
Container(
width: double.infinity,
margin: const EdgeInsets.all(40.0),
padding: const EdgeInsets.all(50.0),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20.0), //Use this code to make rounded corners
color: const Color(0xffCCCCCC),
),
child: const Text('This box has rounded corners!'),
),
The above code is another example to make the corners of the container widget rounded shape. We are adding a 20.0 radius to the corners of container box.
Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(20.0),
),
),
),
The code can be used to add the border radius to the top left corner only. We use topLeft property for that and assign a value to it using Radius.circular().
Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(
bottomLeft: Radius.circular(20.0),
),
),
),
The code can be used to add the border radius to the bottom left corner only.
We use bottomLeft property for that and assign a value to it using Radius.circular().
We use bottomLeft property for that and assign a value to it using Radius.circular().
Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(20.0),
),
),
),
The code can be used to add the border radius to the top right corner only.
We use topRight property for that and assign a value to it using Radius.circular().
We use topRight property for that and assign a value to it using Radius.circular().
Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(
bottomRight: Radius.circular(20.0),
),
),
),
The code can be used to add the border radius to the bottom right corner only.
We use bottomRight property for that and assign a value to it using Radius.circular().
We use bottomRight property for that and assign a value to it using Radius.circular().
Was this helpful?
Similar Posts