How to Filter an Array of Objects in React
When working with data in React, it is often necessary to filter an array of objects. There are many ways to do this, but in this article, we will discuss how to filter an array of objects in React using the native filter() method.
function App() {
// create an array of objects
const users = [
{id: 1, name: 'John', status: 'active'},
{id: 2, name: 'Rusk', status: 'inactive'},
{id: 3, name: 'Erlic', status: 'active'},
{id: 4, name: 'Sara', status: 'active'},
{id: 5, name: 'Kat', status: 'inactive'}
]
// filter array based on active status
const filterd_users = users.filter( user => user.status === 'active' );
return (
<div>
<h3>Active Users</h3>
<ul>
{filterd_users.map(user =>
<li key={user.id}>{user.name}</li>
)}
</ul>
</div>
);
}
Output
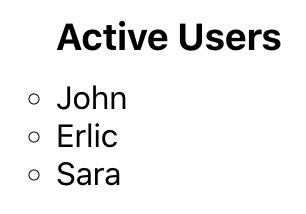
In the above code example, we have created an array of objects called users.
It has three keys in each object - id, name, and status.
We are filtering the user array using the filter() function. We are checking if the status key has value - active and returning the object if matched.
The filter() method takes two arguments: a callback function and an optional object. The callback function is invoked for each element in the array, and the object is used as this value.
The callback function takes three arguments: the value of the element, the index of the element, and the array being filtered.
If the callback function returns true, the element is included in the return array. Otherwise, it's excluded.
If you want to get the users that have inactive status then you can filter the user array as below.
const filterd_inactive_users = users.filter( user => user.status === 'inactive' );
The above code will return the below array in the filtered_inactive_users variable.
const users = [
{id: 2, name: 'Rusk', status: 'inactive'},
{id: 5, name: 'Kat', status: 'inactive'}
]
- Collect books from array of objects and return collection of books as an array
- Javascript ES6 Array Filter
- Finding the max/min value of an attribute in an array of objects
- Get total sum of specif attribute inside of array of objects
- Filter array
- Sort array of objects by key value in Javascript
- Filter an Array using object key value in Javascript