Create a searchable list in React
React is a powerful JavaScript library that helps developers create user interfaces and websites. When it comes to creating a searchable list, React provides a great solution. This article will show you how to create a searchable list in React without using any library.
function App() {
const myData = ["Apple", "Banana", "Orange", "Papaya", "Grapes"];
const [filteredData, setFilteredData] = useState(myData);
function filterData(e) {
const q = e.target.value.toLowerCase();
const filteredRecords = myData.filter( item => item.toLowerCase().includes(q) );
setFilteredData(filteredRecords);
}
return (
<div className="container">
<div className="search_box">
<input type="text" onKeyUp={filterData} className="searchCtrl" placeholder="Search the list" />
</div>
<div className="box">
{filteredData && filteredData.length > 0 ? (
<ul className="list">
{filteredData.map((item, index) =>
<li key={index}>{item}</li>
)}
</ul>
) : <div className="message">Not Data Found</div>
}
</div>
</div>
);
}
Output
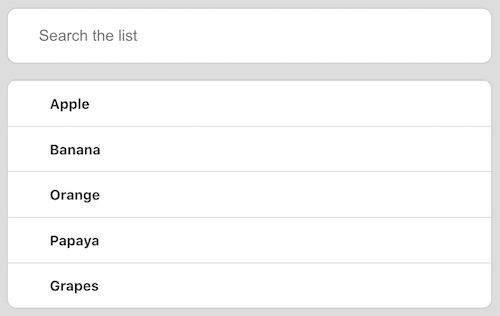
In the above code example, we have created an array called myData that contains multiple items. We are assigning it to filteredData variable that will contain filtered items. When we type something in the input box created in react we call a method that will filter the data and assign new array to filteredData variable.
We are using string items in the previous code example. If you have objects in the array and you want to create a searchable list based on multiple key value pairs then you can use the below code example.
function App() {
const myData = [
{name: "Jack Ham", dept: "Math"},
{name: "Soniya", dept: "Arts"},
{name: "Rick Grimes", dept: "Math"},
{name: "Carol Pam", dept: "Arts"}
];
const [filteredData, setFilteredData] = useState(myData);
function filterData(e) {
const q = e.target.value.toLowerCase();
const filteredRecords = myData.filter( item =>
item.name.toLowerCase().includes(q) || item.dept.toLocaleLowerCase().includes(q)
);
setFilteredData(filteredRecords);
}
return (
<div className="container">
<div className="search_box">
<input type="text" onKeyUp={filterData} className="searchCtrl" placeholder="Search the list" />
</div>
<div className="box">
{filteredData && filteredData.length > 0 ? (
<ul className="list">
{filteredData.map((item, index) =>
<li key={index}>{item.name} ({item.dept})</li>
)}
</ul>
) : <div className="message">Not Data Found</div>
}
</div>
</div>
);
}
We are making out an array of objects called myData searchable here. We have applied a filter on the name and dept keys here. If the input text exists in these key-value it will return that array item else It will show the message "No data found".
The CSS that we are using for the above list is:
.container {
max-width: 500px;
margin: auto;
}
.searchCtrl {
width: 100%;
margin-bottom: 1em;
height: 3.6em;
border: 1px solid #c9c9c9;
border-radius: 10px;
font-size: 1em;
padding: 0 2em;
}
.box {
background-color: #fff;
border: 1px solid #ccc;
border-radius: 10px;
}
.list li {
display: flex;
width: 100%;
border-top: 1px solid #ddd;
padding: 1em 3em;
font-size: .9em;
font-weight: bold;
color: #3b3737;
}
.message {
padding: 1em 2em;
font-size: 1em;
color: #ff0000;
font-weight: bold;
}