javascript
Get index in map React
September 24, 2022
The map() function is a built-in JavaScript function that can be used to iterate over arrays and objects. The map() function can be used with React to track the index of an element in an array. The index can then be used to get the value of the element at that index. The map() function will return a new array with the index of the element as the first element and the value of the element as the second element.
function App() {
const users = [
{id: 1, name: 'John'},
{id: 2, name: 'Rusk'},
{id: 3, name: 'Erlic'}
]
return (
<ul>
{users.map((item, index) =>
<li key={index}>Index is: {index}. User is {item.name}</li>
)}
</ul>
);
}
Output
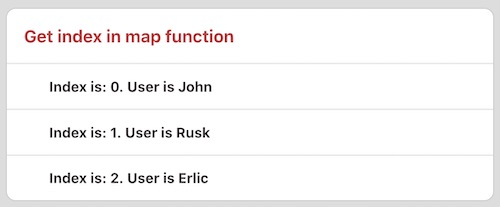
The minimal code that can be used to get the index inside the map() function is:
{users.map((item, index) =>
<li key={index}>Index is: {index}. User is {item.name}</li>
)}
In the map function, you can find the index as its second parameter.
Was this helpful?
Similar Posts