Check if two dictionaries are equal in Python
Dictionaries are a handy data type in Python, that allows you to work with key-value pairs, which can be retrieved either by their key or their value. In this post, we will learn how to check if two dictionaries are equal or not using multiple methods.
dict1 = {"a": 1, "b": 2, "c": 4}
dict2 = {"a": 1, "b": 2, "c": 3}
if dict1 == dict2:
print("The dictionaries are equal")
else:
print("The dictionaries are not equal")
Output
The dictionaries are not equal
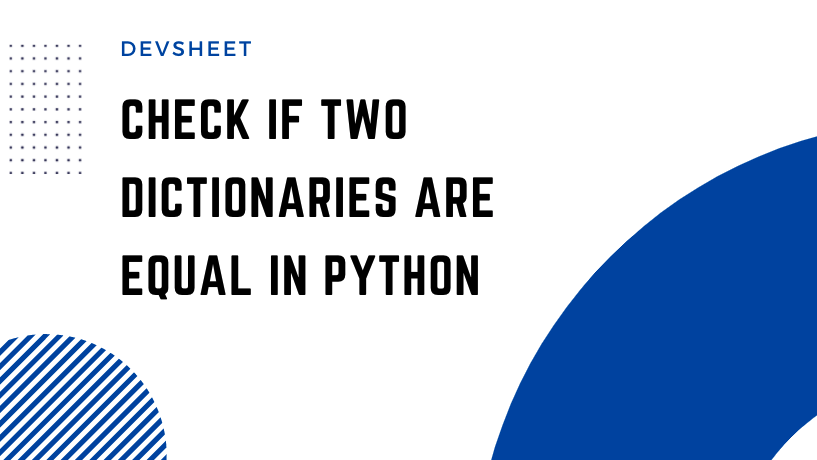
Use == operator to check if the dictionaries are equal
The simplest technique to check if two or multiple dictionaries are equal is by using the == operator in Python. You can create the dictionaries with any of the methods defined in Python and then compare them using the == operator. It will return True the dictionaries are equals and False if not.
Syntax
dict1 == dict2 == dict3
Code example
dict1 = dict(a=1, b=2, c=3)
dict2 = dict(zip(['a', 'b', 'c'], [1, 2, 3]))
dict3 = dict([('b', 2), ('a', 1), ('c', 3)])
dict4 = dict({'b': 2, 'a': 1, 'c': 3})
# compare all dictionaries
if dict1 == dict2 == dict3 == dict4:
print('The dictionaries are equal')
# compare two dictionaries
if dict2 == dict4:
print('The dictionaries are equal')
Output
The dictionaries are equal
The dictionaries are equal
Explanation of the above code example
- Created four dictionaries(dict1, dict2, dict3, dict4) using different methods.
- Using dict1 == dict2 == dict3 == dict4 code syntax we are comparing all the dictionaries. This will return True if all the dictionaries are equal.
- Using dict2 == dict4 code, we are comparing two dictionaries.
- Print message - "The dictionaries are equal" if the dictionaries are equal.
Compare dictionaries using the deepdiff python module
You can install the Python module deepdiff in your project and check the differences between the two dictionaries. We can install the deepdiff module using the below command in your project.
pip install deepdiff
We will be using the DeepDiff() function from the deepdiff module to compare the dictionaries. It will return the dictionary that will contain the information of the difference between the dictionaries.
Syntax
DeepDiff(dict1, dict2)
from deepdiff import DeepDiff
dict1 = {'a': 1, 'b': 2, 'c': 4}
dict2 = {'a': 1, 'c': 3, 'd': 5}
result = DeepDiff(dict1, dict2)
print(result)
Output
{'dictionary_item_added': [root['d']], 'dictionary_item_removed': [root['b']], 'values_changed': {"root['c']": {'new_value': 3, 'old_value': 4}}}
Get common key-value pairs from two dictionaries
We can get the common key-value pairs from two dictionaries and check if those are equal or not. We will be using Python For loop to the common key-value pairs.
- Create two dictionaries that contain some properties.
- Create a blank dictionary that will contain the common key-value pairs.
- In each iteration of For Loop check if the key from the second dictionary exists in the first dictionary. Also, check if the value from both dictionaries is the same for the same key. If this condition is found true, add that key-value pair to the common dictionary variable.
dict1 = {"a": 1, "b": 2, "c": 3}
dict2 = {"a": 3, "b": 2, "c": 3, "d": 4}
common = {}
for key in dict1:
if ((key in dict2) and (dict1[key] == dict2[key])):
common[key] = dict1[key]
# print common key-value pairs
print(common)
Output
{'b': 2, 'c': 3}
- Merge two or multiple dictionaries in Python
- Create equal size chunks from a list in Python
- Python collection - Dictionaries in python
- [Python] Using comprehension expression to convert list of dictionaries to nested dictionary
- Get all values by key name from a list of dictionaries Python
- Convert pandas DataFrame to List of dictionaries python
- Convert JSON string to Python collections - like list, dictionaries