Reverse a string or number in python
If you are using Python to develop your application and have a requirement to reverse a string or number then you can use one of the methods explained in this post.
Reverse a string or number in python
To reverse a string in Python:
- Create a string my_str = "hello".
- Use string[::-1] to reverse the string my_str[::-1].
# Reverse a string
my_str = "hello"
reversed_str = my_str[::-1]
print(reversed_str)
# -> olleh
# Reverse a number
my_num = 12345
reversed_num = str(my_num)[::-1]
print(reversed_num)
# Output - 54321
The above code example shows the fastest way to reverse a Python String or a number. We just pass the [::-1] after the variable name to reverse a string.
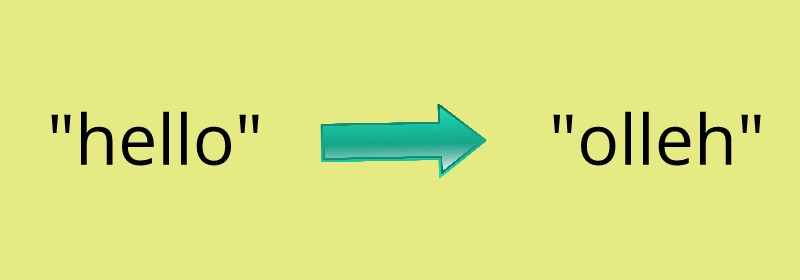
Syntax
Variable[::-1]
Reverse a number in Python
We will use the same syntax here that we are using in the above code example to reverse a number in Python. To reverse a number with the same approach, we need to convert it to string type first and after reversing the number we are converting it back to an int value.
my_num = 34567
result = int( str(my_num)[::-1] )
print(result)
Output
76543
Reverse a String in Python
We can use the slicing to reverse a String in Python. Like numbers, we don't need to convert a String to anything. We can use the slicing of String easily and reverse it with one line of code.
input_str = "reverse this string"
result = input_str[::-1]
print(result)
Output
gnirts siht esrever
Create a Python Function to reverse number or string
def reverse_it(value):
return value[::-1]
#reverse a string
print( reverse_it('triangle') ) # -> elgnairt
# revers a number
print( reverse_it(str(12345)) ) # -> 54321
The given Python code defines a function called "reverse_it" that takes a single parameter called "value". The function returns the reverse of the input value by using slicing notation to access the elements of the value in reverse order.
The slicing notation used in the function is [::-1], which means to start at the end of the value, take every element in reverse order, and stop at the beginning of the value.
The function is then called with two different arguments: a string and an integer converted to a string. When the function is called with the string "triangle", it returns the reverse of the string, which is "elgnairt". When the function is called with the string representation of the integer 12345, it returns the reverse of the integer, which is 54321.
The output of the function is printed using the print() function. The output for the first call to the function is "elgnairt" and the output for the second call to the function is "54321".