Add margin to container in Flutter
In Flutter, you can add margins to a container by using the EdgeInsets class. The EdgeInsets class defines the margins for each side of the container (top, right, bottom, and left).
Container(
margin: EdgeInsets.all(40.0),
child: Text(
'Hello world'
),
),
Add margin to container in Flutter
To add a margin to a Container widget in Flutter:
- Create a container widget using Container().
- Use EdgeInsets to add a margin. eg: Container(margin: const EdgeInsets.all(20.0),).
Here's an example of how to add margins to a container:
Container(
margin: const EdgeInsets.all(16.0),
child: Text('Hello World!'),
),
In the example above, we've added 16.0 pixels of margin to each side of the container by using the EdgeInsets.all constructor.
Add margin to symmetric sides of the Container
You can also use other EdgeInsets constructors to define margins for symmetric sides
(vertically and horizontally) of the container.
For example:
Container(
margin: const EdgeInsets.symmetric(vertical: 16.0),
child: Text('Hello World!'),
)
In this example, we've added 16.0 pixels of vertical margin to the container (i.e., the margin on the top and bottom sides) by using the EdgeInsets.symmetric constructor.
Container(
margin: const EdgeInsets.symmetric(
vertical: 30.0,
horizontal: 40.0,
),
child: Text('Hello World!'),
)
The above code will add a margin of 30px on the vertical sides and a 40px margin on the horizontal sides.
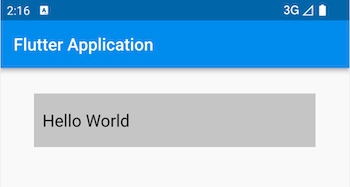
Add margin to the specific sides of the Container
In Flutter, you can add margins to specific sides of a container by using the EdgeInsets class. The EdgeInsets class defines the margins for each side of the container (top, right, bottom, and left). Here's an example of how to add margins to specific sides of a container:
Container(
margin: EdgeInsets.only(left: 16.0),
child: Text('Hello World!'),
);
In the example above, we've added 16.0 pixels of margin to the left side of the container by using the EdgeInsets.only constructor. You can also use other EdgeInsets constructors to define margins for specific sides of the container. For example:
Container(
margin: EdgeInsets.fromLTRB(16.0, 8.0, 24.0, 12.0),
child: Text('Hello World!'),
);
In this example, we've added 16.0 pixels of margin to the left side of the container, 8.0 pixels of margin to the top side, 24.0 pixels of margin to the right side, and 12.0 pixels of margin to the bottom side by using the EdgeInsets.fromLTRB constructor. You can also use the EdgeInsets.symmetric constructor to define margins for multiple sides at once, as shown in the previous answer.