Add padding to container in Flutter
Padding is a useful feature in Flutter which allows developers to set a specific amount of space between the edges of a container and its child elements. Padding helps to visually separate elements and can be used to create more interesting and aesthetically pleasing interfaces. In this tutorial, we will explain how to add padding to a container in Flutter and provide some examples of how to effectively use padding in design.
Add padding to Container in Flutter
To add padding to a Container widget:
- Create a Container widget in Flutter - Container()
- Use padding property to add padding to it - Container(padding: EdgeInsets.all(50.0), child: Text('Hello'))
In the following example, we are adding padding of 50px to each side of the Container widget.
Container(
padding: const EdgeInsets.all(50.0),
child: Text('Hello World'),
),
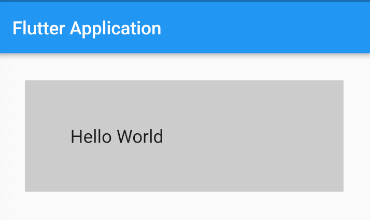
To learn more about the padding property of the Container widget, check the following code examples.
Add Padding to each side of the Container
Adding padding to each side of a container in Flutter is a simple process that involves using the EdgeInsets.all(val) value to the padding property. This property allows developers to specify an exact amount of padding to add to each side of the container.
Container(
padding: const EdgeInsets.all(20.0),
child: Text('Hello World'),
),
The above code example will add a 20px padding to each side of the Container widget.
Add padding to specific sides of the Container
You can also specify different padding values for each side of the Container by using the EdgeInsets.only() method:
Container(
padding: const EdgeInsets.only(
top: 10,
bottom: 20,
left: 30,
right: 40,
),
child: Text('Hello World'),
)
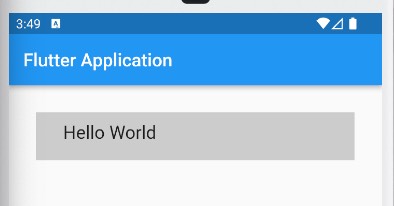
This code example creates a Container widget with the child widget 'Hello World' and sets the padding of the Container to 10px on the top, 20px on the bottom, 30px on the left, and 40px on the right. The padding is set using the EdgeInsets.only method.
Add padding to the symmetric sides of the Container
To add padding to the symmetric sides of a Container in Flutter, use the EdgeInsets.symmetric() constructor to create a symmetric EdgeInsets object. Pass this EdgeInsets object to the padding parameter of the Container. For example:
Container(
padding: const EdgeInsets.symmetric(
vertical: 20.0,
horizontal: 10.0,
),
child: Text('Hello World'),
)
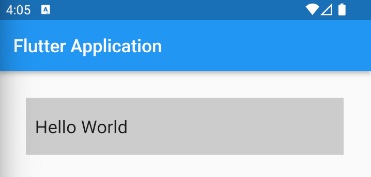
This Flutter code creates a Container widget which contains a Text widget with the text "Hello World". The Container widget has a padding of 20.0 pixels on the top and bottom, and 10.0 pixels on the left and right. This will create a margin around the Text widget.
Conclusion
Padding in Flutter is used to add space between the content and the edges of a container. It is a way to provide spacing and make the content of the container more visually appealing and easier to read.
In Flutter, the Container widget is a very versatile and commonly used widget that can be used to display a variety of UI elements. Adding padding to a Container is a common way to ensure that the content inside the Container is spaced out appropriately.
Padding can also be used to make a Container or any other widget more touchable. For example, if you have a button widget, adding padding to the button widget will increase the size of the touchable area and make it easier to tap on a mobile device.
Overall, using padding in Flutter containers and other widgets is an essential technique to make your UI more visually appealing and improve user experience.