Delete the first column in a Pandas DataFrame
A Pandas DataFrame is a two-dimensional data structure that can hold data of various types. You can think of it like a spreadsheet, with columns and rows. Sometimes you may want to delete a column from a DataFrame. This can be done using the .drop() method.
import pandas as pd
# creating a DataFrame
df = pd.DataFrame({
'name': ['Rachel', 'Jacob', 'Dom', 'Syral', 'Naboi'],
'city': ['Japan', 'NewYork', 'London', 'Itly', 'France'],
'status': ['active', 'inactive', 'active', 'active', 'inactive'],
'salary': [3000, 5000, 2000, 7000, 4000]
})
print("Original DataFrame:")
print(df)
# delete first column(name) from DataFrame - df
df.drop(columns=df.columns[0], axis=1, inplace=True)
print("DataFrame after deletion:")
print(df)
Output
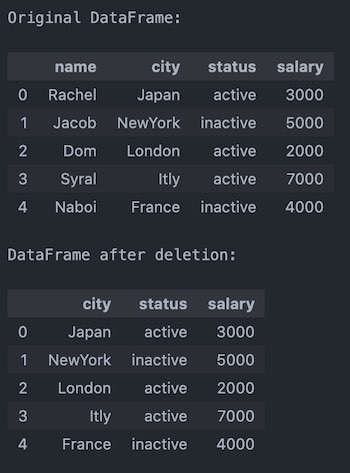
Method 1: Delete first column of DataFrame using drop() function
The drop() function is used to delete the first column of a Pandas Dataframe. This is useful if you want to get rid of a column that is not needed or is causing problems.
Syntax
df.drop(columns=df.columns[0], axis=1, inplace=True)
We use axis=1 for column and axis=0 for rows.
Full code example
import pandas as pd
# creating a DataFrame
df = pd.DataFrame({
'Fruit' : ['Apple', 'Orange', 'Grapes', 'Banana', 'Papaya'],
'Quantity' : [50, 30, 60, 45, 50],
'Price' : [100, 390, 500, 460, 600],
'Color': ['Red', 'Orange', 'Green', 'Yellow', 'Yellow']
})
print("Original DataFrame:")
print(df)
# delete first column(Fruit) from DataFrame - df
df.drop(columns=df.columns[0], axis=1, inplace=True)
print("DataFrame after deletion:")
print(df)
Output
Original DataFrame:
+----+---------+------------+---------+---------+
| | Fruit | Quantity | Price | Color |
|----+---------+------------+---------+---------|
| 0 | Apple | 50 | 100 | Red |
| 1 | Orange | 30 | 390 | Orange |
| 2 | Grapes | 60 | 500 | Green |
| 3 | Banana | 45 | 460 | Yellow |
| 4 | Papaya | 50 | 600 | Yellow |
+----+---------+------------+---------+---------+
DataFrame after deletion:
+----+------------+---------+---------+
| | Quantity | Price | Color |
|----+------------+---------+---------|
| 0 | 50 | 100 | Red |
| 1 | 30 | 390 | Orange |
| 2 | 60 | 500 | Green |
| 3 | 45 | 460 | Yellow |
| 4 | 50 | 600 | Yellow |
+----+------------+---------+---------+
1. The code first imports the pandas library, which provides a DataFrame object type that can be used to store data in a tabular format.
2. Next, a DataFrame object is created using a dictionary. The dictionary contains four key-value pairs, with the keys being the column names ('Fruit', 'Quantity', 'Price', and 'Color') and the values being the data for each column.
3. The code then prints the original DataFrame.
4. Next, the first column of the DataFrame is dropped using the .drop() method. The columns parameter is set to df.columns[0] in order to specify which column to drop, and the axis parameter is set to 1 in order to indicate that the column is being dropped from the DataFrame. The inplace parameter is set to True in order to modify the DataFrame in place.
If you want to delete one or multiple columns from DataFrame using column names, you can check the below article.
Delete one or multiple columns from Pandas Dataframe
Method 2: Delete the first column of DataFrame using iloc
You can delete the first column from a DataFrame using the iloc method. iloc is integer-based indexing for selection by position in Pandas DataFrame.
Syntax
df = df.iloc[:, 1:]
Code example
import pandas as pd
# creating a DataFrame
df = pd.DataFrame({
'Fruit' : ['Apple', 'Orange', 'Grapes', 'Banana', 'Papaya'],
'Quantity' : [50, 30, 60, 45, 50],
'Price' : [100, 390, 500, 460, 600],
'Color': ['Red', 'Orange', 'Green', 'Yellow', 'Yellow']
})
print("Original DataFrame:")
print(df)
# delete first column(Fruit) from DataFrame - df
df = df.iloc[:, 1:]
print("DataFrame after deletion:")
print(df)
Output
Original DataFrame:
+----+---------+------------+---------+---------+
| | Fruit | Quantity | Price | Color |
|----+---------+------------+---------+---------|
| 0 | Apple | 50 | 100 | Red |
| 1 | Orange | 30 | 390 | Orange |
| 2 | Grapes | 60 | 500 | Green |
| 3 | Banana | 45 | 460 | Yellow |
| 4 | Papaya | 50 | 600 | Yellow |
+----+---------+------------+---------+---------+
DataFrame after deletion:
+----+------------+---------+---------+
| | Quantity | Price | Color |
|----+------------+---------+---------|
| 0 | 50 | 100 | Red |
| 1 | 30 | 390 | Orange |
| 2 | 60 | 500 | Green |
| 3 | 45 | 460 | Yellow |
| 4 | 50 | 600 | Yellow |
+----+------------+---------+---------+
Method 3: Use del keyword to delete the first column of Pandas DataFrame
If you want to remove the first column of a Pandas DataFrame, you can use the del keyword. This will delete the column from the DataFrame, and you can access the remaining data using the column names.
Syntax
del df[df.columns[0]]
Code example
import pandas as pd
# creating a DataFrame
df = pd.DataFrame({
'Fruit' : ['Apple', 'Orange', 'Grapes', 'Banana', 'Papaya'],
'Quantity' : [50, 30, 60, 45, 50],
'Price' : [100, 390, 500, 460, 600],
'Color': ['Red', 'Orange', 'Green', 'Yellow', 'Yellow']
})
print("Original DataFrame:")
print(df)
# delete first column(Fruit) from DataFrame - df
del df[df.columns[0]]
print("DataFrame after deletion:")
print(df)
Output
Original DataFrame:
+----+---------+------------+---------+---------+
| | Fruit | Quantity | Price | Color |
|----+---------+------------+---------+---------|
| 0 | Apple | 50 | 100 | Red |
| 1 | Orange | 30 | 390 | Orange |
| 2 | Grapes | 60 | 500 | Green |
| 3 | Banana | 45 | 460 | Yellow |
| 4 | Papaya | 50 | 600 | Yellow |
+----+---------+------------+---------+---------+
DataFrame after deletion:
+----+------------+---------+---------+
| | Quantity | Price | Color |
|----+------------+---------+---------|
| 0 | 50 | 100 | Red |
| 1 | 30 | 390 | Orange |
| 2 | 60 | 500 | Green |
| 3 | 45 | 460 | Yellow |
| 4 | 50 | 600 | Yellow |
+----+------------+---------+---------+
- Pandas - Delete,Remove,Drop, column from pandas DataFrame
- [Pandas] Add new column to DataFrame based on existing column
- Change column orders using column names list - Pandas Dataframe
- Delete one or multiple columns from Pandas Dataframe
- Pandas - Delete multiple rows from DataFrame using index list
- Reorder dataframe columns using column names in pandas
- Get a value from DataFrame row using index and column name in pandas