Pandas - Change rows order of a DataFrame using index list
Sorting or reordering Dataframe rows is quite a common operation. DataFrame provides the sort method for that, but this method sorts a DataFrame based on the values in its columns. In this post, we will reorder the rows based on the index list.
import pandas as pd
# create a dictionary
students = {
"name": ["Tom", "John", "Rick", "Sneha", "Johnson"],
"score": [90, 80, 98, 99, 82],
"subjects": ["Math", "Physics", "Chemistry", "English", "Data Structure"]
}
# create the dataframe from dictionary
df = pd.DataFrame(students, index=['a', 'b', 'c', 'd', 'e'])
print("Original DataFrame: ")
print(df)
#change rows orders using index list
df = df.reindex(['d', 'a', 'e', 'b', 'c'])
print("After ordering using row index:")
print(df)
Output
Original DataFrame:
+----+---------+---------+----------------+
| | name | score | subjects |
|----+---------+---------+----------------|
| a | Tom | 90 | Math |
| b | John | 80 | Physics |
| c | Rick | 98 | Chemistry |
| d | Sneha | 99 | english |
| e | Johnson | 82 | Data Structure |
+----+---------+---------+----------------+
After ordering using row index:
+----+---------+---------+----------------+
| | name | score | subjects |
|----+---------+---------+----------------|
| d | Sneha | 99 | English |
| a | Tom | 90 | Math |
| e | Johnson | 82 | Data Structure |
| b | John | 80 | Physics |
| c | Rick | 98 | Chemistry |
+----+---------+---------+----------------+
If you are using the Pandas library in your Python project then you must be using DataFrames to perform specific tasks on large data. You can sort columns and rows by value using the sort() function. But sometimes it is required to change the orders of rows using the DataFrame index. We can arrange them in ascending and descending order easily but if you want to sort them using a Python List that contains the indexes of Dataframe as its items. We use DataFrame.reindex() function to reorder the rows using the index list.
Explanation of the above code example
1. Created a dictionary named students that contains multiple key-value pairs. We will be using keys as column names and values as rows of Dataframe. The values in the student's dictionary are in the form of a List.
2. Created a DataFrame from the above dictionary. We are also assigning custom indexes to the DataFrame. We are using the below code syntax to do that.
df = pd.DataFrame(students, index=['a', 'b', 'c', 'd', 'e'])
3. Using reindex() function of Pandas Dataframe to change the order of rows.
df.reindex(['d', 'a', 'e', 'b', 'c'])
Rearrange rows order based on default indexes list
If you're working with data in Pandas, there may be times when you need to rearrange the order of your rows. Maybe you want to sort your data by a certain column, or you want to group rows together based on a certain index. Whatever the reason, it's easy to rearrange rows in Pandas using the default index list.
Code example
import pandas as pd
# create a dictionary
students = {
"name": ["Tom", "John", "Rick", "Sneha", "Johnson"],
"score": [90, 80, 98, 99, 82],
"subjects": ["Math", "Physics", "Chemistry", "english", "Data Structure"]
}
df = pd.DataFrame(students)
print("Original DataFrame")
print(df)
#change rows orders using index list - made from default indexes
df = df.reindex([3, 4, 0, 1, 2])
print("After ordering using row index")
print(df)
Output
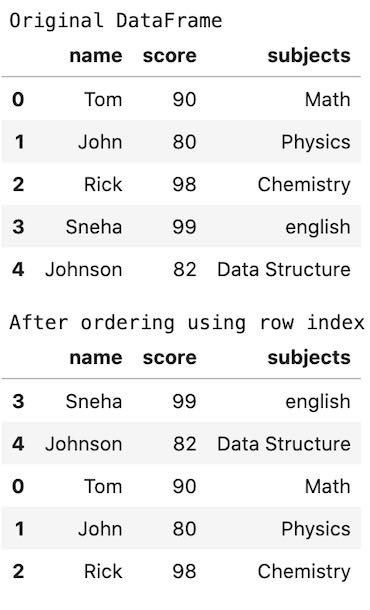
- Pandas - Delete multiple rows from DataFrame using index list
- Change column orders using column names list - Pandas Dataframe
- Get a column rows as a List in Pandas Dataframe
- Pandas - Get index values of a DataFrame as a List
- Get a value from DataFrame row using index and column name in pandas
- Change the name of columns in a pandas dataframe
- Change column values condition based in Pandas DataFrame