flutter
Round Container with Image in Flutter
September 25, 2022
This tutorial will show you how to create a round container with an image in Flutter. This is a great way to display a user profile image or list item images.
Container(
width: 200.0,
height: 200.0,
margin: const EdgeInsets.all(50.0),
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(
Radius.circular(100.0),
),
image: DecorationImage(
image: NetworkImage('https://flutter.github.io/assets-for-api-docs/assets/widgets/owl-2.jpg'),
fit: BoxFit.cover,
),
),
),
Output
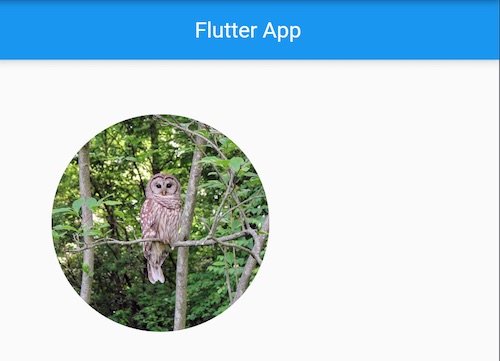
The code above creates a Container widget with a width and height of 200.0. It also has a margin of 50.0 on all sides.
The decoration property is set to a BoxDecoration with a borderRadius of 100.0.
The image property is set to a DecorationImage with a NetworkImage.
The fit property is set to BoxFit.cover.
Full code example:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter App',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatelessWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Flutter App"),
),
body: Container(
width: 200.0,
height: 200.0,
margin: const EdgeInsets.all(50.0),
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(
Radius.circular(100.0),
),
image: DecorationImage(
image: NetworkImage('https://flutter.github.io/assets-for-api-docs/assets/widgets/owl-2.jpg'),
fit: BoxFit.cover,
),
),
),
);
}
}
Alternative of the above code using ClipRRect
Container(
width: 200.0,
height: 200.0,
margin: const EdgeInsets.all(50.0),
child: ClipRRect(
borderRadius: BorderRadius.circular(100.0),
child: Image.network(
'https://flutter.github.io/assets-for-api-docs/assets/widgets/owl-2.jpg',
fit: BoxFit.cover,
),
)
),
Was this helpful?
Similar Posts